Tutorial-04: Layered Plots¶
On top of the information in tutorial-03, this adds a plot of the
phonon mean free path at each band and q-point in the simulation.
The colour in this case corresponds to the band index, although a range of
more informative variables can be projected onto the colour axis with
add_projected_waterfall
. This colouring can also be removed by specifying a
single colour rather than a colourmap. This contains much the same information
as the broadening in tutorial-02, with smaller values showing more scattering
and lower lattice thermal conductivity.
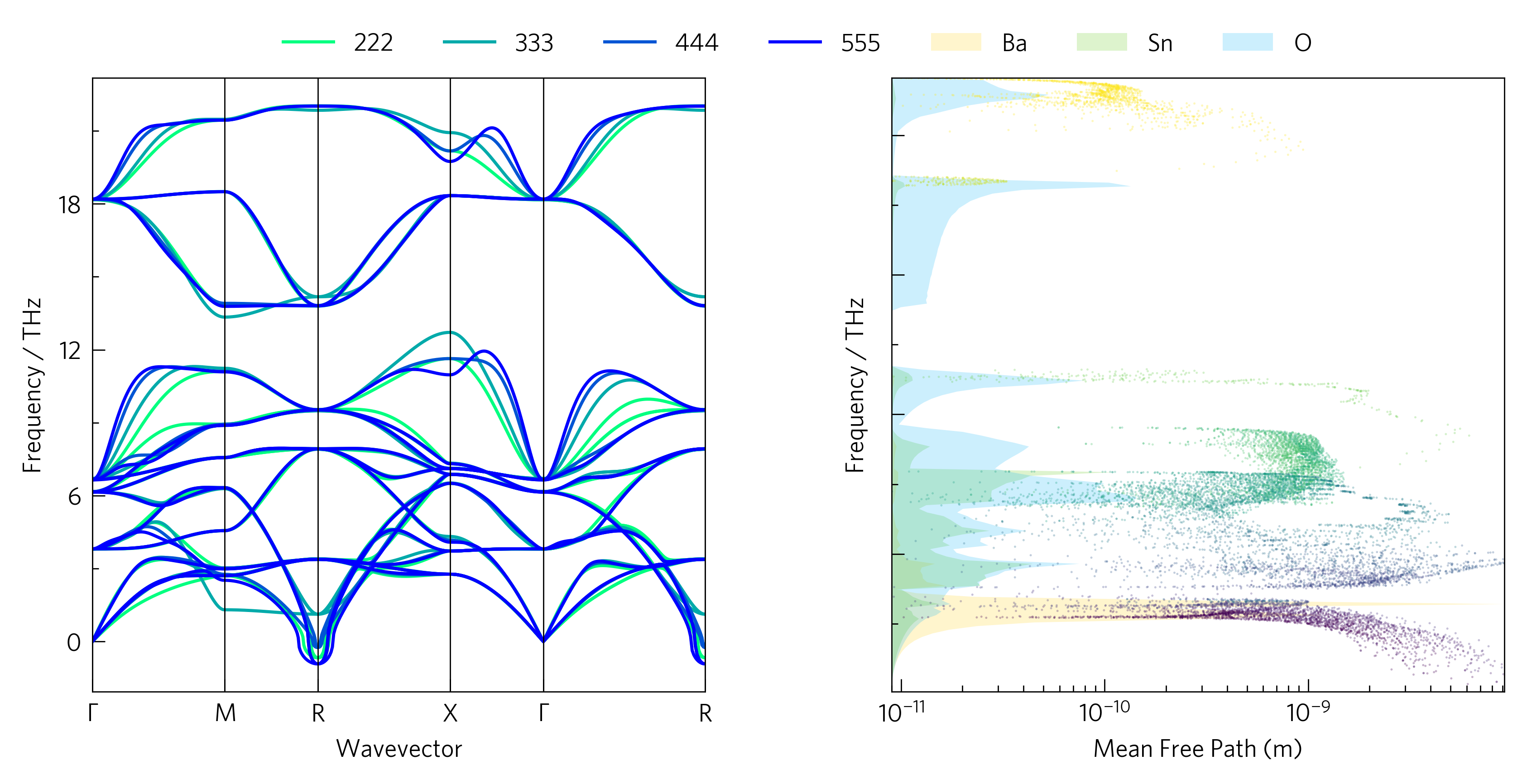
As well as plotting graphs on neighbouring axes, it can be informative to plot them on the same axes to highlight links, for example how the mean free paths of phonons in BaSnO3 dip where Ba has a strong contribution to the DoS, or how a phonon dispersion converges with supercell size. Although this can start to make scripts more complicated, and is not yet supported by the CLI (with the exception of phonon convergence), it is often worth the effort.
The python version of this code is:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | #!/usr/bin/env python3
import tp
scs = '222 333 444 555'.split()
pfiles = ['../data/basno3/band-{}.yaml'.format(s) for s in scs]
kfile = '../data/basno3/kappa-m363636.hdf5'
dfile = '../data/basno3/projected_dos.dat'
poscar = '../data/basno3/POSCAR'
direction = 'avg'
temperature = 300
waterfall = 'mean_free_path'
quantities = ['waterfall', waterfall]
colour = 'winter_r'
colours = {'Ba': '#ffcf06',
'Sn': '#59c605',
'O': '#00b1f7'}
cmap = 'viridis'
# You can ignore down to line 23!
from os import path
if not path.isfile(kfile) or (path.getsize(kfile) < 1024*1024*100):
raise Exception('File not found, please use get-data.sh in the folder above.')
# Stop ignoring!
# Axes
fig, ax, add_legend = tp.axes.small.two_h()
# Load
dispersions = [tp.data.load.phonopy_dispersion(f) for f in pfiles]
kappa = tp.data.load.phono3py(kfile, quantities=quantities)
dos = tp.data.load.phonopy_dos(dfile, poscar=poscar)
# Plot
tp.plot.phonons.add_multi(ax[0], dispersions, colour=colour, label=scs)
tp.plot.frequency.format_waterfall(ax[1], kappa, waterfall, direction=direction,
temperature=temperature, invert=True)
tp.plot.frequency.add_dos(ax[1], dos, colour=colours, scale=True, main=False,
alpha=0.6, line=False, invert=True)
tp.plot.frequency.add_waterfall(ax[1], kappa, waterfall, colour=cmap,
direction=direction, temperature=temperature,
invert=True)
# Formatting
tp.plot.utilities.set_locators(ax[1], x='log', y='linear')
axlabels = tp.settings.labels()
ax[1].set_xlabel(axlabels['mean_free_path'])
ax[1].set_ylabel(axlabels['frequency'])
add_legend()
# Save
fig.savefig('tutorial-04.png')
|
Axes (line 29)¶
Here we use two_h
. h stands for horizontal, and there is a
corresponding vertical set of axes, two_v
. They also come with
space for colourbars, by adding _colourbars
to their names.
Load (line 32)¶
We need lists of files here, we’ve used list comprehensions to generate the file names and load them, but you could also use something like:
from glob import glob
pfiles = glob('band-*.yaml')
Although in that case, you would also need to define the labels.
Plot (lines 38-44)¶
add_dos
and add_cumkappa
have the arguments main
and
scale
, which enable them to be used on the same axes as plots with
other axis scales. main
causes the axes ticks, labels and limits to
be set for the plot, so turning it off doesn’t interfere with the
current configuration. scale
scales the data to the axes, rather
than the axes to the data, so everything is visible. If both main
and scale
are set, the y-axis (or x, if invert
) is scaled to
percent.
It can look better to have the waterfall plot above the DoS, but the
DoS should be scaled to the waterfall axes. Therefore, there is a
function, format_waterfall
, which sets the scale without plotting
the waterfall plot. Running format_waterfall
, then add_dos
,
then add_waterfall
solves this, however it doesn’t work properly if
line=True
.
Formatting (lines 48-51)¶
Setting invert
removes the y-axis labels and shortens the x-axis
one in anticipation of being a DoS-style set of axes, but this can be
reversed with some helper functions: set_locators
sets the axis
scales and tick locators for each axis, and also has a DoS argument,
which removes the ticks and tick labels and the y axis label, while all
the default labels can be accessed with settings.labels
. These will
be covered more in Tutorial-05.